obspy.core - Core classes of ObsPy
This package contains common methods and classes for ObsPy. It includes the
Stream
, Trace
,
UTCDateTime
, Stats
classes and methods for reading
seismogram
files.
- copyright:
The ObsPy Development Team (devs@obspy.org)
- license:
GNU Lesser General Public License, Version 3 (https://www.gnu.org/copyleft/lesser.html)
Waveform Data
Summary
Seismograms of various formats (e.g. SAC, MiniSEED, GSE2, SEISAN, Q, etc.) can
be imported into a Stream
object using the
read()
function.
Streams are list-like objects which contain multiple
Trace
objects, i.e. gap-less continuous time series
and related header/meta information.
Each Trace object has the attribute data
pointing to a NumPy
ndarray
of the actual time series and the attribute stats
which contains all meta information in a dict-like
Stats
object. Both attributes starttime
and
endtime
of the Stats object are
UTCDateTime
objects.
A multitude of helper methods are attached to
Stream
and Trace
objects
for handling and modifying the waveform data.
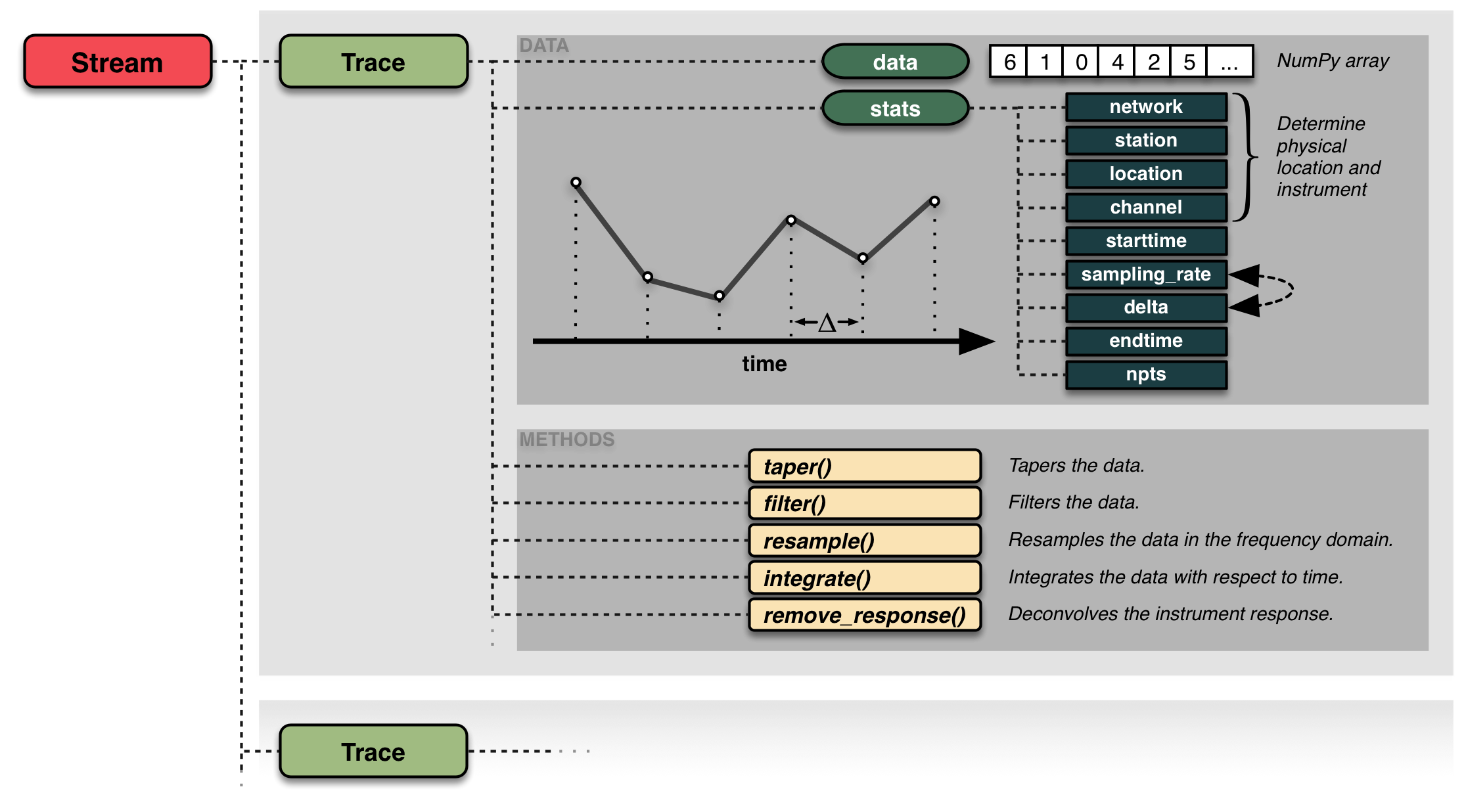
Example
A Stream
with an example seismogram can be created
by calling read()
without any arguments.
Local files can be read by specifying the filename, files stored on http
servers (e.g. at https://examples.obspy.org) can be read by specifying their
URL. For details and supported formats see the documentation of
read()
.
>>> from obspy import read
>>> st = read()
>>> print(st)
3 Trace(s) in Stream:
BW.RJOB..EHZ | 2009-08-24T00:20:03.000000Z - ... | 100.0 Hz, 3000 samples
BW.RJOB..EHN | 2009-08-24T00:20:03.000000Z - ... | 100.0 Hz, 3000 samples
BW.RJOB..EHE | 2009-08-24T00:20:03.000000Z - ... | 100.0 Hz, 3000 samples
>>> tr = st[0]
>>> print(tr)
BW.RJOB..EHZ | 2009-08-24T00:20:03.000000Z - ... | 100.0 Hz, 3000 samples
>>> tr.data
array([ 0. , 0.00694644, 0.07597424, ..., 1.93449584,
0.98196204, 0.44196924])
>>> print(tr.stats)
network: BW
station: RJOB
location:
channel: EHZ
starttime: 2009-08-24T00:20:03.000000Z
endtime: 2009-08-24T00:20:32.990000Z
sampling_rate: 100.0
delta: 0.01
npts: 3000
calib: 1.0
...
>>> tr.stats.starttime
UTCDateTime(2009, 8, 24, 0, 20, 3)
Event Metadata
Event metadata are handled in a hierarchy of classes closely modelled after the
de-facto standard format QuakeML.
See the IPython notebooks mentioned in the ObsPy Tutorial for
more detailed usage examples. See
read_events()
and
Catalog.write()
for supported
formats.
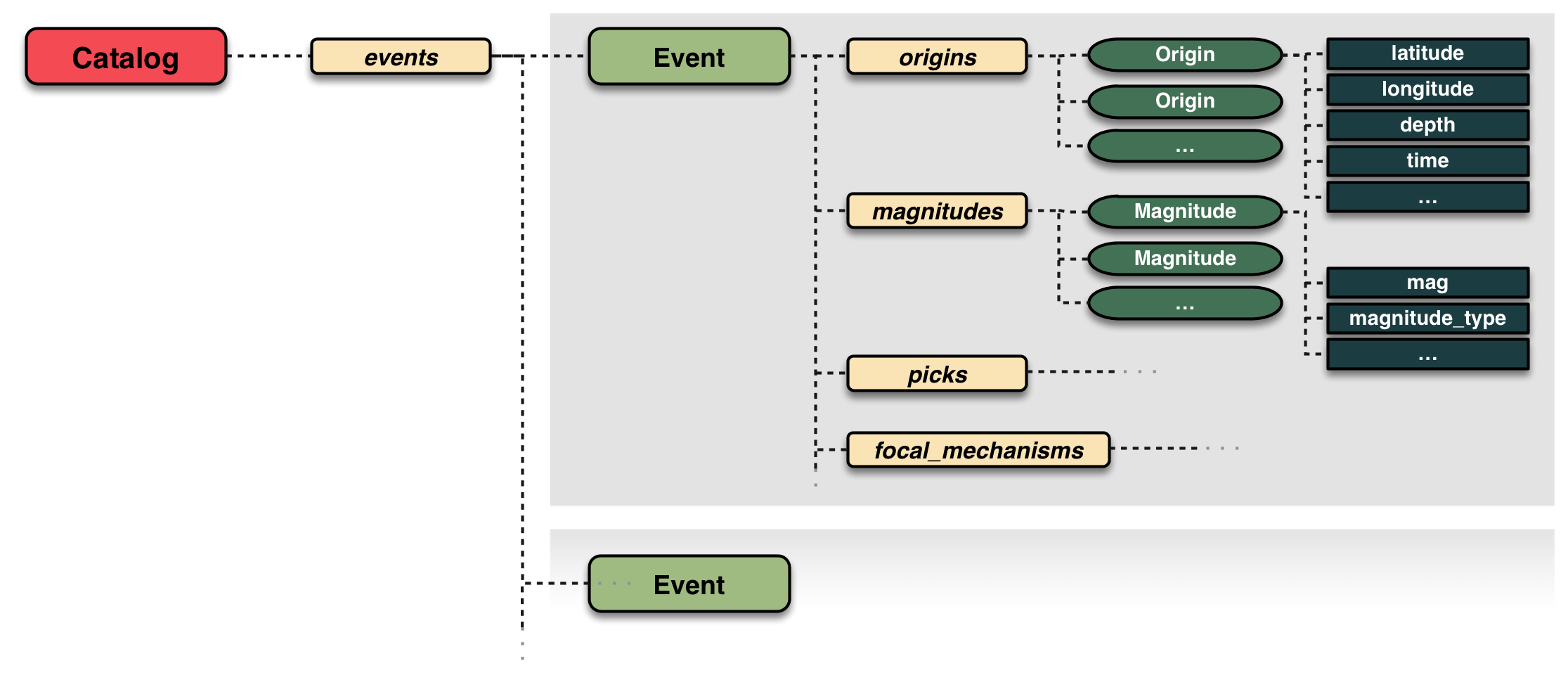
Station Metadata
Station metadata are handled in a hierarchy of classes closely modelled after
the de-facto standard format
FDSN StationXML which was developed as a
human readable XML replacement for Dataless SEED.
See obspy.core.inventory
for more details. See
read_inventory()
and
Inventory.write()
for
supported formats.
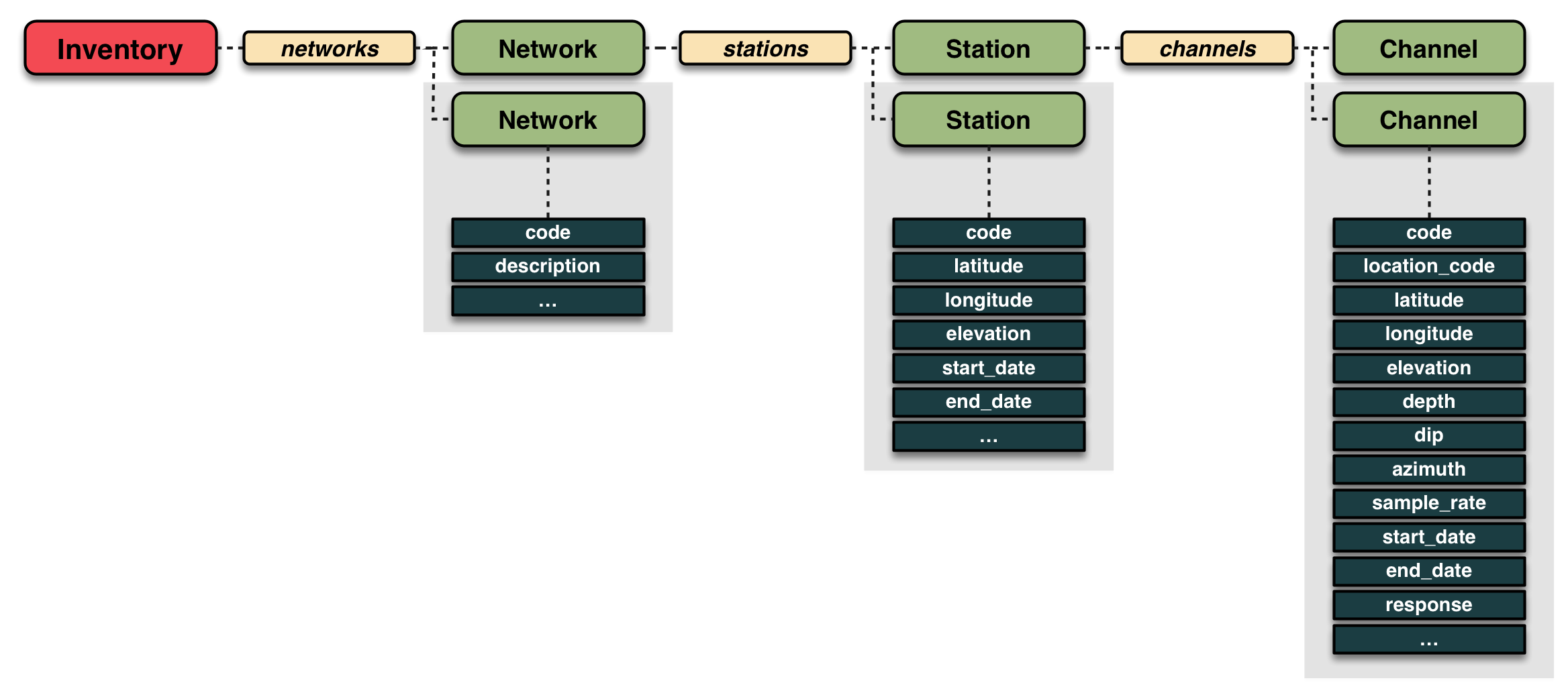
Classes & Functions
Read waveform files into an ObsPy |
|
List like object of multiple ObsPy |
|
An object containing data of a continuous series, such as a seismic trace. |
|
A container for additional header information of a ObsPy |
|
A UTC-based datetime object. |
|
Read event files into an ObsPy |
|
This class serves as a container for |
|
The class Event describes a seismic event which does not necessarily need to be a tectonic earthquake. |
|
Function to read inventory files. |
|
The root object of the |
Modules
Module for handling ObsPy |
|
Module containing a UTC-based datetime class. |
|
Module handling event metadata |
|
Module for handling station metadata |
|
Various utilities for ObsPy |
|
Tools for creating and merging previews. |